Using List and Grid in Android -Recycleview
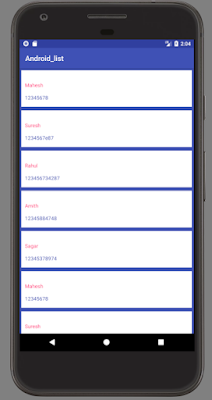
- The displacements in List or Grid is Very common in Mobile Development,
- the user can sea collection of list and scroll through them,
- The collection of things can be list or Grid,
- to show elements like Grid type or list type we can Use Recycleview.
RecyclerView:
The RecyclerView widget is a more advanced and flexible version of ListView. This widget is a container for displaying large data sets that can be scrolled very efficiently by maintaining a limited number of views.
Using RecyclerView :
- The recycle view supports the display collection of Data
- it is a moderate version of Listview and Gridview from Android Framework.
- Recycleview Uses ViewHolder to store a reference of view for One entry.
- ViewHolder is static Inner class in Adapter it holds reference of Relevant Views
Gradel Dependency for Recyclview:
Add following dependency to gradle
compile "com.android.support:recyclerview-v7:26.1.0"
.
Adapters:
- Adapter manages the data model and adapts into the into the individual entry in the widge
- it extends RecyclerView.Adapter
android Recyclview development step by Step:
step-1: (create list-item-view )
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout
android:layout_margin="5dp" android:layout_marginTop="5dp" android:orientation="vertical" xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="wrap_content">
<TextView android:gravity="bottom" android:text="Mahesh" android:id="@+id/txt_Name" android:textColor="@color/colorAccent" android:layout_width="match_parent" android:layout_height="50dp" />
<TextView android:gravity="center_vertical" android:text="1234567891" android:id="@+id/txt_number" android:textColor="@color/colorPrimary" android:layout_width="match_parent" android:layout_height="50dp" />
</LinearLayout>
Step-2:(Create UserModel):
Create Model
public class UserModel {
public String _Name;
public String _Number;
public UserModel(String _Name, String _Number) {
this._Name = _Name;
this._Number = _Number;
}
public String get_Name() {
return _Name;
}
public void set_Name(String _Name) {
this._Name = _Name;
}
public String get_Number() {
return _Number;
}
public void set_Number(String _Number) {
this._Number = _Number;
}
}
Step-3 : (Create Adapter Class with View Holder)
Crate Adapter For class
public class Adapter extends RecyclerView.Adapter<Adapter.ViewHolder> {
ArrayList<UserModel> userData=new ArrayList<>();
public Adapter(ArrayList<UserModel> userData) {
this.userData = userData;
}
@Override public ViewHolder onCreateViewHolder(ViewGroup parent, int viewType) {
LayoutInflater inflater = LayoutInflater.from(parent.getContext());
View v= inflater.inflate(R.layout.listitem_row,parent,false);
ViewHolder holder=new ViewHolder(v);
return holder;
}
@Override public void onBindViewHolder(ViewHolder holder, int position) {
/* Get User Info Based On Position */ UserModel curentUser= userData.get(position);
holder._txtName.setText(curentUser._Name);
holder._txtNumber.setText(curentUser._Number);
}
@Override public int getItemCount() {
/* Get Count from Data */ return userData.size();
}
public class ViewHolder extends RecyclerView.ViewHolder{
public TextView _txtName;
public TextView _txtNumber;
public ViewHolder(View v) {
super(v);
_txtName= v.findViewById(R.id.txt_Name);
_txtNumber= v.findViewById(R.id.txt_number);
}
}
}
step-4: (Add recyview in Main layout):
<android.support.constraint.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android" android:background="@color/colorPrimary" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context="in.arula.android_list.MainActivity">
<android.support.v7.widget.RecyclerView android:id="@+id/my_recycler_view" android:layout_width="match_parent" android:layout_height="match_parent">
</android.support.v7.widget.RecyclerView>
</android.support.constraint.ConstraintLayout>
Step-5 (Write Code in Main-Activity):
public class MainActivity extends AppCompatActivity {
ArrayList<UserModel> userData=new ArrayList<>();
private RecyclerView recyclerView;
private RecyclerView.Adapter mAdapter;
private RecyclerView.LayoutManager layoutManager;
@Override protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
/* Add data to User List */ Adddata();
recyclerView = (RecyclerView) findViewById(R.id.my_recycler_view);
// use this setting to // improve performance if you know that changes // in content do not change the layout size // of the RecyclerView
recyclerView.setHasFixedSize(true);
// use a linear layout manager layoutManager = new LinearLayoutManager(this);
recyclerView.setLayoutManager(layoutManager);
mAdapter = new Adapter(userData);
recyclerView.setAdapter(mAdapter);
}
private void Adddata() {
userData.add(new UserModel("Mahesh","12345678"));
userData.add(new UserModel("Suresh","1234567e87"));
userData.add(new UserModel("Rahul","123456734287"));
userData.add(new UserModel("Amith","12345884748"));
userData.add(new UserModel("Sagar","12345378974"));
userData.add(new UserModel("Mahesh","12345678"));
userData.add(new UserModel("Suresh","1234567e87"));
userData.add(new UserModel("Rahul","123456734287"));
userData.add(new UserModel("Amith","12345884748"));
userData.add(new UserModel("Sagar","12345378974"));
}
}
No comments